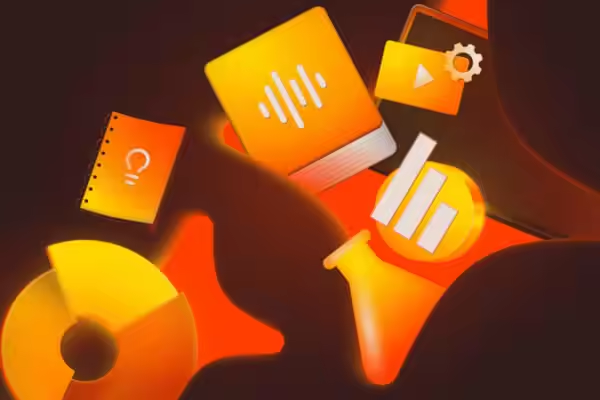
wpautop ( $text, $br = true )
wpautop – 这个函数用来在WordPress的文本周围添加段落标签。它负责自动将双倍的换行符转换为HTML段落: 该函数经常被用来格式化WordPress中的文章和页面的内容。
用段落元素替换双倍换行符。
一组正则表达式替换符用于标识用换行符格式化的文本,并用HTML段落标记替换双换行符。除非“$br”设置为“0”或“false”,否则转换后剩余的换行符将变为“
”标记。
function wpautop( $text, $br = true ) { $pre_tags = array(); if ( trim( $text ) === '' ) { return ''; } // Just to make things a little easier, pad the end. $text = $text . "n"; /* * Pre tags shouldn't be touched by autop. * Replace pre tags with placeholders and bring them back after autop. */ if ( strpos( $text, '<pre' ) !== false ) { $text_parts = explode( '</pre>', $text ); $last_part = array_pop( $text_parts ); $text = ''; $i = 0; foreach ( $text_parts as $text_part ) { $start = strpos( $text_part, '<pre' ); // Malformed HTML? if ( false === $start ) { $text .= $text_part; continue; } $name = "<pre wp-pre-tag-$i></pre>"; $pre_tags[ $name ] = substr( $text_part, $start ) . '</pre>'; $text .= substr( $text_part, 0, $start ) . $name; $i++; } $text .= $last_part; } // Change multiple <br>'s into two line breaks, which will turn into paragraphs. $text = preg_replace( '|<brs*/?>s*<brs*/?>|', "nn", $text ); $allblocks = '(?:table|thead|tfoot|caption|col|colgroup|tbody|tr|td|th|div|dl|dd|dt|ul|ol|li|pre|form|map|area|blockquote|address|math|style|p|h[1-6]|hr|fieldset|legend|section|article|aside|hgroup|header|footer|nav|figure|figcaption|details|menu|summary)'; // Add a double line break above block-level opening tags. $text = preg_replace( '!(<' . $allblocks . '[s/>])!', "nn$1", $text ); // Add a double line break below block-level closing tags. $text = preg_replace( '!(</' . $allblocks . '>)!', "$1nn", $text ); // Add a double line break after hr tags, which are self closing. $text = preg_replace( '!(<hrs*?/?>)!', "$1nn", $text ); // Standardize newline characters to "n". $text = str_replace( array( "rn", "r" ), "n", $text ); // Find newlines in all elements and add placeholders. $text = wp_replace_in_html_tags( $text, array( "n" => ' <!-- wpnl --> ' ) ); // Collapse line breaks before and after <option> elements so they don't get autop'd. if ( strpos( $text, '<option' ) !== false ) { $text = preg_replace( '|s*<option|', '<option', $text ); $text = preg_replace( '|</option>s*|', '</option>', $text ); } /* * Collapse line breaks inside <object> elements, before <param> and <embed> elements * so they don't get autop'd. */ if ( strpos( $text, '</object>' ) !== false ) { $text = preg_replace( '|(<object[^>]*>)s*|', '$1', $text ); $text = preg_replace( '|s*</object>|', '</object>', $text ); $text = preg_replace( '%s*(</?(?:param|embed)[^>]*>)s*%', '$1', $text ); } /* * Collapse line breaks inside <audio> and <video> elements, * before and after <source> and <track> elements. */ if ( strpos( $text, '<source' ) !== false || strpos( $text, '<track' ) !== false ) { $text = preg_replace( '%([<[](?:audio|video)[^>]]*[>]])s*%', '$1', $text ); $text = preg_replace( '%s*([<[]/(?:audio|video)[>]])%', '$1', $text ); $text = preg_replace( '%s*(<(?:source|track)[^>]*>)s*%', '$1', $text ); } // Collapse line breaks before and after <figcaption> elements. if ( strpos( $text, '<figcaption' ) !== false ) { $text = preg_replace( '|s*(<figcaption[^>]*>)|', '$1', $text ); $text = preg_replace( '|</figcaption>s*|', '</figcaption>', $text ); } // Remove more than two contiguous line breaks. $text = preg_replace( "/nn+/", "nn", $text ); // Split up the contents into an array of strings, separated by double line breaks. $paragraphs = preg_split( '/ns*n/', $text, -1, PREG_SPLIT_NO_EMPTY ); // Reset $text prior to rebuilding. $text = ''; // Rebuild the content as a string, wrapping every bit with a <p>. foreach ( $paragraphs as $paragraph ) { $text .= '<p>' . trim( $paragraph, "n" ) . "</p>n"; } // Under certain strange conditions it could create a P of entirely whitespace. $text = preg_replace( '|<p>s*</p>|', '', $text ); // Add a closing <p> inside <div>, <address>, or <form> tag if missing. $text = preg_replace( '!<p>([^<]+)</(div|address|form)>!', '<p>$1</p></$2>', $text ); // If an opening or closing block element tag is wrapped in a <p>, unwrap it. $text = preg_replace( '!<p>s*(</?' . $allblocks . '[^>]*>)s*</p>!', '$1', $text ); // In some cases <li> may get wrapped in <p>, fix them. $text = preg_replace( '|<p>(<li.+?)</p>|', '$1', $text ); // If a <blockquote> is wrapped with a <p>, move it inside the <blockquote>. $text = preg_replace( '|<p><blockquote([^>]*)>|i', '<blockquote$1><p>', $text ); $text = str_replace( '</blockquote></p>', '</p></blockquote>', $text ); // If an opening or closing block element tag is preceded by an opening <p> tag, remove it. $text = preg_replace( '!<p>s*(</?' . $allblocks . '[^>]*>)!', '$1', $text ); // If an opening or closing block element tag is followed by a closing <p> tag, remove it. $text = preg_replace( '!(</?' . $allblocks . '[^>]*>)s*</p>!', '$1', $text ); // Optionally insert line breaks. if ( $br ) { // Replace newlines that shouldn't be touched with a placeholder. $text = preg_replace_callback( '/<(script|style|svg).*?</\1>/s', '_autop_newline_preservation_helper', $text ); // Normalize <br> $text = str_replace( array( '<br>', '<br/>' ), '<br />', $text ); // Replace any new line characters that aren't preceded by a <br /> with a <br />. $text = preg_replace( '|(?<!<br />)s*n|', "<br />n", $text ); // Replace newline placeholders with newlines. $text = str_replace( '<WPPreserveNewline />', "n", $text ); } // If a <br /> tag is after an opening or closing block tag, remove it. $text = preg_replace( '!(</?' . $allblocks . '[^>]*>)s*<br />!', '$1', $text ); // If a <br /> tag is before a subset of opening or closing block tags, remove it. $text = preg_replace( '!<br />(s*</?(?:p|li|div|dl|dd|dt|th|pre|td|ul|ol)[^>]*>)!', '$1', $text ); $text = preg_replace( "|n</p>$|", '</p>', $text ); // Replace placeholder <pre> tags with their original content. if ( ! empty( $pre_tags ) ) { $text = str_replace( array_keys( $pre_tags ), array_values( $pre_tags ), $text ); } // Restore newlines in all elements. if ( false !== strpos( $text, '<!-- wpnl -->' ) ) { $text = str_replace( array( ' <!-- wpnl --> ', '<!-- wpnl -->' ), "n", $text ); } return $text; }
要使用` get_users
`函数获取所有用户列表,可以按照以下步骤进行:
1. 使用` get_users
`函数调用获取用户列表:
$users = get_users();
2. 您可以按需使用参数来过滤结果。例如,您可以通过角色、用户ID、用户登录名等过滤用户列表。以下是一个根据用户角色为过滤条件的示例:
$users = get_users( array( 'role' => 'subscriber' // 将角色名称替换为您要过滤的角色 ) );
在上述示例中,将` role
`参数设置为所需的角色名称来过滤用户列表。
3. 您可以使用循环遍历获取的用户列表,并访问每个用户的属性。例如,以下示例将显示每个用户的用户名和电子邮件地址:
foreach( $users as $user ) { echo '用户名:' . $user->user_login . ', 电子邮件:' . $user->user_email . ; }
在上述示例中,通过` $user->user_login
`和` $user->user_email
`访问每个用户的用户名和电子邮件地址。
请注意,` get_users
`函数默认返回所有用户,并可以根据需要使用更多参数进行过滤。您可以参阅WordPress官方文档中的` get_users
`函数文档,了解更多可用参数和用法示例。
总结起来,使用` get_users
`函数获取所有用户列表的步骤是:
get_users
`函数获取用户列表。在WordPress中,可以使用WP_PLUGIN_DIR和WP_PLUGIN_URL常量来定义插件的目录路径和URL。
1. `WP_PLUGIN_DIR`:这是一个常量,用于定义插件的目录路径(文件系统路径)。您可以使用以下代码在插件文件中访问该常量:
$plugin_dir = WP_PLUGIN_DIR . '/your-plugin-folder/';
在上述代码中,将"your-plugin-folder"替换为您插件的实际文件夹名称。使用该常量,您可以获取插件文件的完整路径。
2. `WP_PLUGIN_URL`:这是一个常量,用于定义插件的URL(用于在网页上访问插件文件)。以下是一个使用该常量的示例:
$plugin_url = WP_PLUGIN_URL . '/your-plugin-folder/';
同样,请将"your-plugin-folder"替换为您插件的实际文件夹名称。使用该常量,您可以获取插件在网页上的完整URL。
请注意,`WP_PLUGIN_DIR`和`WP_PLUGIN_URL`常量在WordPress版本2.6之后引入。从WordPress 5.5版本开始,这两个常量被标记为过时(deprecated),因为WordPress更倾向于使用新的插件文件结构。如果您正在开发新插件,建议使用新的插件文件结构和相关函数。
在新的插件文件结构中,可以使用以下函数来获取插件的目录路径和URL:
- `plugin_dir_path()`:获取插件目录路径。
- `plugin_dir_url()`:获取插件URL。
这些函数会自动将插件的版本、多站点和SSL等考虑因素纳入计算。
总结起来,使用`WP_PLUGIN_DIR`和`WP_PLUGIN_URL`常量定义插件的目录和URL的方法是:
$plugin_dir = WP_PLUGIN_DIR . '/your-plugin-folder/'; $plugin_url = WP_PLUGIN_URL . '/your-plugin-folder/';
但请注意,这两个常量已被标记为过时,建议使用新的插件文件结构和相关函数来获取插件的路径和URL。
使用PHP在WordPress中添加自定义功能可以通过以下方式实现:
下面是一个实操示例。
要在WordPress中添加自定义功能,可以按照以下步骤使用PHP编写并添加自定义功能:
// 添加自定义功能示例 // 1. 创建自定义短代码 function custom_shortcode() { return '这是我的自定义短代码内容'; } add_shortcode('custom', 'custom_shortcode'); // 2. 自定义小工具 function custom_widget() { echo '这是我的自定义小工具内容'; } register_widget('custom_widget'); // 3. 自定义菜单 function custom_menu() { register_nav_menu('custom-menu', '自定义菜单'); } add_action('after_setup_theme', 'custom_menu'); // 4. 自定义页面模板 function custom_page_template() { /* Template Name: 自定义模板 */ // 自定义模板的内容和样式 }
请注意,修改主题文件可以在主题更新时丢失,因此建议在进行任何更改之前备份functions.php文件。此外,为避免不必要的错误和冲突,建议在添加自定义功能前先了解WordPress开发文档和最佳实践,以确保正确、安全地实现所需的自定义功能。
使用 do_action
函数可以触发一个钩子函数。do_action
函数的参数与要触发的钩子函数的参数相同。
例如,触发save_post钩子函数的代码如下:
do_action( 'save_post', $post_ID, $post );
这里,$post_ID
和 $post
是传递给钩子函数的参数。
使用 wp_get_current_user
获取当前登录用户的信息:
$current_user = wp_get_current_user(); // 获取当前用户的ID $user_id = $current_user->ID; // 获取当前用户的用户名 $user_login = $current_user->user_login; // 获取当前用户的邮箱 $user_email = $current_user->user_email; // 获取当前用户的显示名称 $display_name = $current_user->display_name;