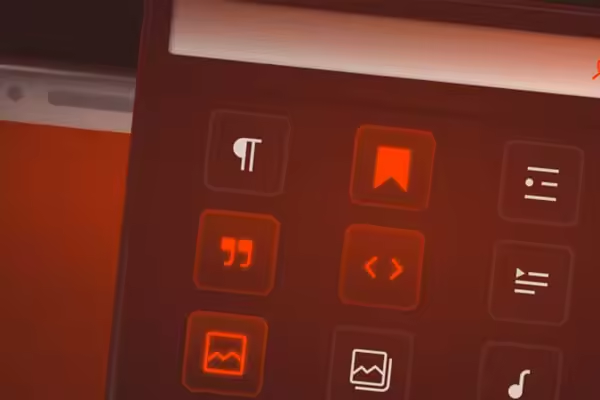
activate_plugin ( $plugin, $redirect = '', $network_wide = false, $silent = false )
activate_plugin: 這個函式用於啟用WordPress中的一個外掛。它執行一些檢查,以確保該外掛可以被啟用,然後在WordPress的選項表中新增一個條目,表明該外掛已經啟用。
在一個”sandbox”中嘗試啟用外掛,成功後重定向。
已經被啟用的外掛不會再嘗試被啟用。
它的工作方式是,在嘗試包含外掛檔案之前,將重定向設定為錯誤。如果外掛失敗了,那麼重定向將不會被成功資訊所覆蓋。同時,選項不會被更新,啟用鉤也不會在外掛出錯時被呼叫。
應該注意的是,下面的程式碼在任何情況下都不會真正防止檔案內的錯誤。這段程式碼不應該被用在其他地方來複制”sandbox”,它使用重定向來工作。{@source 1}
如果發現任何錯誤或輸出文字,那麼將被捕獲以確保成功重定向將更新錯誤重定向。
function activate_plugin( $plugin, $redirect = '', $network_wide = false, $silent = false ) { $plugin = plugin_basename( trim( $plugin ) ); if ( is_multisite() && ( $network_wide || is_network_only_plugin( $plugin ) ) ) { $network_wide = true; $current = get_site_option( 'active_sitewide_plugins', array() ); $_GET['networkwide'] = 1; // Back compat for plugins looking for this value. } else { $current = get_option( 'active_plugins', array() ); } $valid = validate_plugin( $plugin ); if ( is_wp_error( $valid ) ) { return $valid; } $requirements = validate_plugin_requirements( $plugin ); if ( is_wp_error( $requirements ) ) { return $requirements; } if ( $network_wide && ! isset( $current[ $plugin ] ) || ! $network_wide && ! in_array( $plugin, $current, true ) ) { if ( ! empty( $redirect ) ) { // We'll override this later if the plugin can be included without fatal error. wp_redirect( add_query_arg( '_error_nonce', wp_create_nonce( 'plugin-activation-error_' . $plugin ), $redirect ) ); } ob_start(); // Load the plugin to test whether it throws any errors. plugin_sandbox_scrape( $plugin ); if ( ! $silent ) { /** * Fires before a plugin is activated. * * If a plugin is silently activated (such as during an update), * this hook does not fire. * * @since 2.9.0 * * @param string $plugin Path to the plugin file relative to the plugins directory. * @param bool $network_wide Whether to enable the plugin for all sites in the network * or just the current site. Multisite only. Default false. */ do_action( 'activate_plugin', $plugin, $network_wide ); /** * Fires as a specific plugin is being activated. * * This hook is the "activation" hook used internally by register_activation_hook(). * The dynamic portion of the hook name, `$plugin`, refers to the plugin basename. * * If a plugin is silently activated (such as during an update), this hook does not fire. * * @since 2.0.0 * * @param bool $network_wide Whether to enable the plugin for all sites in the network * or just the current site. Multisite only. Default false. */ do_action( "activate_{$plugin}", $network_wide ); } if ( $network_wide ) { $current = get_site_option( 'active_sitewide_plugins', array() ); $current[ $plugin ] = time(); update_site_option( 'active_sitewide_plugins', $current ); } else { $current = get_option( 'active_plugins', array() ); $current[] = $plugin; sort( $current ); update_option( 'active_plugins', $current ); } if ( ! $silent ) { /** * Fires after a plugin has been activated. * * If a plugin is silently activated (such as during an update), * this hook does not fire. * * @since 2.9.0 * * @param string $plugin Path to the plugin file relative to the plugins directory. * @param bool $network_wide Whether to enable the plugin for all sites in the network * or just the current site. Multisite only. Default false. */ do_action( 'activated_plugin', $plugin, $network_wide ); } if ( ob_get_length() > 0 ) { $output = ob_get_clean(); return new WP_Error( 'unexpected_output', __( 'The plugin generated unexpected output.' ), $output ); } ob_end_clean(); } return null; }
要使用` get_users
`函式獲取所有使用者列表,可以按照以下步驟進行:
1. 使用` get_users
`函式呼叫獲取使用者列表:
$users = get_users();
2. 您可以按需使用引數來過濾結果。例如,您可以通過角色、使用者ID、使用者登入名等過濾使用者列表。以下是一個根據使用者角色為過濾條件的示例:
$users = get_users( array( 'role' => 'subscriber' // 將角色名稱替換為您要過濾的角色 ) );
在上述示例中,將` role
`引數設定為所需的角色名稱來過濾使用者列表。
3. 您可以使用迴圈遍歷獲取的使用者列表,並訪問每個使用者的屬性。例如,以下示例將顯示每個使用者的使用者名稱和電子郵件地址:
foreach( $users as $user ) { echo '使用者名稱:' . $user->user_login . ', 電子郵件:' . $user->user_email . ; }
在上述示例中,通過` $user->user_login
`和` $user->user_email
`訪問每個使用者的使用者名稱和電子郵件地址。
請注意,` get_users
`函式預設返回所有使用者,並可以根據需要使用更多引數進行過濾。您可以參閱WordPress官方文件中的` get_users
`函式文件,瞭解更多可用引數和用法示例。
總結起來,使用` get_users
`函式獲取所有使用者列表的步驟是:
get_users
`函式獲取使用者列表。在WordPress中,可以使用WP_PLUGIN_DIR和WP_PLUGIN_URL常量來定義外掛的目錄路徑和URL。
1. `WP_PLUGIN_DIR`:這是一個常量,用於定義外掛的目錄路徑(檔案系統路徑)。您可以使用以下程式碼在外掛檔案中訪問該常量:
$plugin_dir = WP_PLUGIN_DIR . '/your-plugin-folder/';
在上述程式碼中,將"your-plugin-folder"替換為您外掛的實際資料夾名稱。使用該常量,您可以獲取外掛檔案的完整路徑。
2. `WP_PLUGIN_URL`:這是一個常量,用於定義外掛的URL(用於在網頁上訪問外掛檔案)。以下是一個使用該常量的示例:
$plugin_url = WP_PLUGIN_URL . '/your-plugin-folder/';
同樣,請將"your-plugin-folder"替換為您外掛的實際資料夾名稱。使用該常量,您可以獲取外掛在網頁上的完整URL。
請注意,`WP_PLUGIN_DIR`和`WP_PLUGIN_URL`常量在WordPress版本2.6之後引入。從WordPress 5.5版本開始,這兩個常量被標記為過時(deprecated),因為WordPress更傾向於使用新的外掛檔案結構。如果您正在開發新外掛,建議使用新的外掛檔案結構和相關函式。
在新的外掛檔案結構中,可以使用以下函式來獲取外掛的目錄路徑和URL:
- `plugin_dir_path()`:獲取外掛目錄路徑。
- `plugin_dir_url()`:獲取外掛URL。
這些函式會自動將外掛的版本、多站點和SSL等考慮因素納入計算。
總結起來,使用`WP_PLUGIN_DIR`和`WP_PLUGIN_URL`常量定義外掛的目錄和URL的方法是:
$plugin_dir = WP_PLUGIN_DIR . '/your-plugin-folder/'; $plugin_url = WP_PLUGIN_URL . '/your-plugin-folder/';
但請注意,這兩個常量已被標記為過時,建議使用新的外掛檔案結構和相關函式來獲取外掛的路徑和URL。
使用PHP在WordPress中新增自定義功能可以通過以下方式實現:
下面是一個實操示例。
要在WordPress中新增自定義功能,可以按照以下步驟使用PHP編寫並新增自定義功能:
// 新增自定義功能示例 // 1. 建立自定義短程式碼 function custom_shortcode() { return '這是我的自定義短程式碼內容'; } add_shortcode('custom', 'custom_shortcode'); // 2. 自定義小工具 function custom_widget() { echo '這是我的自定義小工具內容'; } register_widget('custom_widget'); // 3. 自定義選單 function custom_menu() { register_nav_menu('custom-menu', '自定義選單'); } add_action('after_setup_theme', 'custom_menu'); // 4. 自定義頁面模板 function custom_page_template() { /* Template Name: 自定義模板 */ // 自定義模板的內容和樣式 }
請注意,修改主題檔案可以在主題更新時丟失,因此建議在進行任何更改之前備份functions.php檔案。此外,為避免不必要的錯誤和衝突,建議在新增自定義功能前先了解WordPress開發文件和最佳實踐,以確保正確、安全地實現所需的自定義功能。
使用 do_action
函式可以觸發一個鉤子函式。do_action
函式的引數與要觸發的鉤子函式的引數相同。
例如,觸發save_post鉤子函式的程式碼如下:
do_action( 'save_post', $post_ID, $post );
這裡,$post_ID
和 $post
是傳遞給鉤子函式的引數。
使用 wp_get_current_user
獲取當前登入使用者的資訊:
$current_user = wp_get_current_user(); // 獲取當前使用者的ID $user_id = $current_user->ID; // 獲取當前使用者的使用者名稱 $user_login = $current_user->user_login; // 獲取當前使用者的郵箱 $user_email = $current_user->user_email; // 獲取當前使用者的顯示名稱 $display_name = $current_user->display_name;