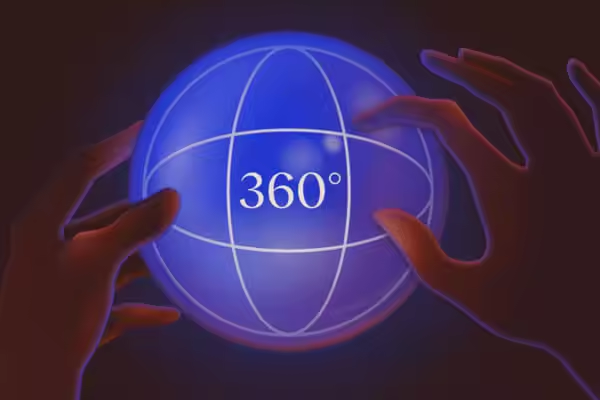
image_add_caption ( $html, $id, $caption, $title, $align, $url, $size, $alt = '' )
image_add_caption: 這個函式用於為圖片新增標題。
在編輯器中新增帶有標題的影象短碼。
function image_add_caption( $html, $id, $caption, $title, $align, $url, $size, $alt = '' ) { /** * Filters the caption text. * * Note: If the caption text is empty, the caption shortcode will not be appended * to the image HTML when inserted into the editor. * * Passing an empty value also prevents the {@see 'image_add_caption_shortcode'} * Filters from being evaluated at the end of image_add_caption(). * * @since 4.1.0 * * @param string $caption The original caption text. * @param int $id The attachment ID. */ $caption = apply_filters( 'image_add_caption_text', $caption, $id ); /** * Filters whether to disable captions. * * Prevents image captions from being appended to image HTML when inserted into the editor. * * @since 2.6.0 * * @param bool $bool Whether to disable appending captions. Returning true from the filter * will disable captions. Default empty string. */ if ( empty( $caption ) || apply_filters( 'disable_captions', '' ) ) { return $html; } $id = ( 0 < (int) $id ) ? 'attachment_' . $id : ''; if ( ! preg_match( '/width=["']([0-9]+)/', $html, $matches ) ) { return $html; } $width = $matches[1]; $caption = str_replace( array( "rn", "r" ), "n", $caption ); $caption = preg_replace_callback( '/<[a-zA-Z0-9]+(?: [^<>]+>)*/', '_cleanup_image_add_caption', $caption ); // Convert any remaining line breaks to <br />. $caption = preg_replace( '/[ nt]*n[ t]*/', '<br />', $caption ); $html = preg_replace( '/(class=["'][^'"]*)align(none|left|right|center)s?/', '$1', $html ); if ( empty( $align ) ) { $align = 'none'; } $shcode = '' . $html . ' ' . $caption . ''; /** * Filters the image HTML markup including the caption shortcode. * * @since 2.6.0 * * @param string $shcode The image HTML markup with caption shortcode. * @param string $html The image HTML markup. */ return apply_filters( 'image_add_caption_shortcode', $shcode, $html ); }
要使用` get_users
`函式獲取所有使用者列表,可以按照以下步驟進行:
1. 使用` get_users
`函式呼叫獲取使用者列表:
$users = get_users();
2. 您可以按需使用引數來過濾結果。例如,您可以通過角色、使用者ID、使用者登入名等過濾使用者列表。以下是一個根據使用者角色為過濾條件的示例:
$users = get_users( array( 'role' => 'subscriber' // 將角色名稱替換為您要過濾的角色 ) );
在上述示例中,將` role
`引數設定為所需的角色名稱來過濾使用者列表。
3. 您可以使用迴圈遍歷獲取的使用者列表,並訪問每個使用者的屬性。例如,以下示例將顯示每個使用者的使用者名稱和電子郵件地址:
foreach( $users as $user ) { echo '使用者名稱:' . $user->user_login . ', 電子郵件:' . $user->user_email . ; }
在上述示例中,通過` $user->user_login
`和` $user->user_email
`訪問每個使用者的使用者名稱和電子郵件地址。
請注意,` get_users
`函式預設返回所有使用者,並可以根據需要使用更多引數進行過濾。您可以參閱WordPress官方文件中的` get_users
`函式文件,瞭解更多可用引數和用法示例。
總結起來,使用` get_users
`函式獲取所有使用者列表的步驟是:
get_users
`函式獲取使用者列表。在WordPress中,可以使用WP_PLUGIN_DIR和WP_PLUGIN_URL常量來定義外掛的目錄路徑和URL。
1. `WP_PLUGIN_DIR`:這是一個常量,用於定義外掛的目錄路徑(檔案系統路徑)。您可以使用以下程式碼在外掛檔案中訪問該常量:
$plugin_dir = WP_PLUGIN_DIR . '/your-plugin-folder/';
在上述程式碼中,將"your-plugin-folder"替換為您外掛的實際資料夾名稱。使用該常量,您可以獲取外掛檔案的完整路徑。
2. `WP_PLUGIN_URL`:這是一個常量,用於定義外掛的URL(用於在網頁上訪問外掛檔案)。以下是一個使用該常量的示例:
$plugin_url = WP_PLUGIN_URL . '/your-plugin-folder/';
同樣,請將"your-plugin-folder"替換為您外掛的實際資料夾名稱。使用該常量,您可以獲取外掛在網頁上的完整URL。
請注意,`WP_PLUGIN_DIR`和`WP_PLUGIN_URL`常量在WordPress版本2.6之後引入。從WordPress 5.5版本開始,這兩個常量被標記為過時(deprecated),因為WordPress更傾向於使用新的外掛檔案結構。如果您正在開發新外掛,建議使用新的外掛檔案結構和相關函式。
在新的外掛檔案結構中,可以使用以下函式來獲取外掛的目錄路徑和URL:
- `plugin_dir_path()`:獲取外掛目錄路徑。
- `plugin_dir_url()`:獲取外掛URL。
這些函式會自動將外掛的版本、多站點和SSL等考慮因素納入計算。
總結起來,使用`WP_PLUGIN_DIR`和`WP_PLUGIN_URL`常量定義外掛的目錄和URL的方法是:
$plugin_dir = WP_PLUGIN_DIR . '/your-plugin-folder/'; $plugin_url = WP_PLUGIN_URL . '/your-plugin-folder/';
但請注意,這兩個常量已被標記為過時,建議使用新的外掛檔案結構和相關函式來獲取外掛的路徑和URL。
使用PHP在WordPress中新增自定義功能可以通過以下方式實現:
下面是一個實操示例。
要在WordPress中新增自定義功能,可以按照以下步驟使用PHP編寫並新增自定義功能:
// 新增自定義功能示例 // 1. 建立自定義短程式碼 function custom_shortcode() { return '這是我的自定義短程式碼內容'; } add_shortcode('custom', 'custom_shortcode'); // 2. 自定義小工具 function custom_widget() { echo '這是我的自定義小工具內容'; } register_widget('custom_widget'); // 3. 自定義選單 function custom_menu() { register_nav_menu('custom-menu', '自定義選單'); } add_action('after_setup_theme', 'custom_menu'); // 4. 自定義頁面模板 function custom_page_template() { /* Template Name: 自定義模板 */ // 自定義模板的內容和樣式 }
請注意,修改主題檔案可以在主題更新時丟失,因此建議在進行任何更改之前備份functions.php檔案。此外,為避免不必要的錯誤和衝突,建議在新增自定義功能前先了解WordPress開發文件和最佳實踐,以確保正確、安全地實現所需的自定義功能。
使用 do_action
函式可以觸發一個鉤子函式。do_action
函式的引數與要觸發的鉤子函式的引數相同。
例如,觸發save_post鉤子函式的程式碼如下:
do_action( 'save_post', $post_ID, $post );
這裡,$post_ID
和 $post
是傳遞給鉤子函式的引數。
使用 wp_get_current_user
獲取當前登入使用者的資訊:
$current_user = wp_get_current_user(); // 獲取當前使用者的ID $user_id = $current_user->ID; // 獲取當前使用者的使用者名稱 $user_login = $current_user->user_login; // 獲取當前使用者的郵箱 $user_email = $current_user->user_email; // 獲取當前使用者的顯示名稱 $display_name = $current_user->display_name;