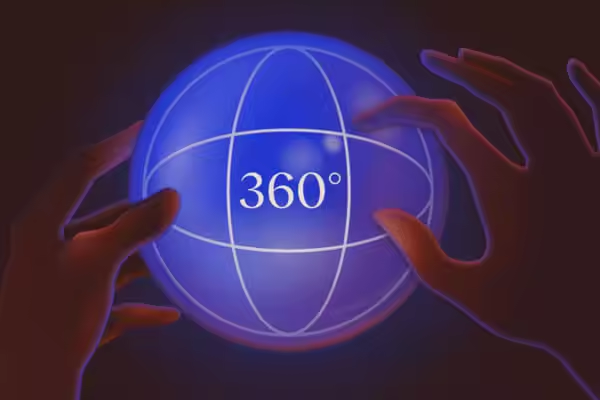
wp_schedule_event ( $timestamp, $recurrence, $hook, $args = array(), $wp_error = false )
wp_schedule_event: 這是一個WordPress的函式,用來安排一個重複發生的事件。它允許你指定一個時間間隔和一個將在該間隔內執行的回撥函式。例如,你可以用這個函式來安排一個指令碼每小時執行一次來執行某個任務。
安排一個經常性的事件。
安排一個鉤子,它將由WordPress在指定的時間間隔內觸發。如果預定的時間已過,當有人訪問你的WordPress網站時,該動作將被觸發。
迴圈事件的有效值是”每小時”、”每天”和”每天兩次”。這些可以使用wp_get_schedules()中的{@see ‘cron_schedules’}過濾器來擴充套件。
使用wp_next_scheduled()來防止重複的事件。
使用wp_schedule_single_event()來安排一個非週期性事件。
function wp_schedule_event( $timestamp, $recurrence, $hook, $args = array(), $wp_error = false ) { // Make sure timestamp is a positive integer. if ( ! is_numeric( $timestamp ) || $timestamp <= 0 ) { if ( $wp_error ) { return new WP_Error( 'invalid_timestamp', __( 'Event timestamp must be a valid Unix timestamp.' ) ); } return false; } $schedules = wp_get_schedules(); if ( ! isset( $schedules[ $recurrence ] ) ) { if ( $wp_error ) { return new WP_Error( 'invalid_schedule', __( 'Event schedule does not exist.' ) ); } return false; } $event = (object) array( 'hook' => $hook, 'timestamp' => $timestamp, 'schedule' => $recurrence, 'args' => $args, 'interval' => $schedules[ $recurrence ]['interval'], ); /** This filter is documented in wp-includes/cron.php */ $pre = apply_filters( 'pre_schedule_event', null, $event, $wp_error ); if ( null !== $pre ) { if ( $wp_error && false === $pre ) { return new WP_Error( 'pre_schedule_event_false', __( 'A plugin prevented the event from being scheduled.' ) ); } if ( ! $wp_error && is_wp_error( $pre ) ) { return false; } return $pre; } /** This filter is documented in wp-includes/cron.php */ $event = apply_filters( 'schedule_event', $event ); // A plugin disallowed this event. if ( ! $event ) { if ( $wp_error ) { return new WP_Error( 'schedule_event_false', __( 'A plugin disallowed this event.' ) ); } return false; } $key = md5( serialize( $event->args ) ); $crons = _get_cron_array(); $crons[ $event->timestamp ][ $event->hook ][ $key ] = array( 'schedule' => $event->schedule, 'args' => $event->args, 'interval' => $event->interval, ); uksort( $crons, 'strnatcasecmp' ); return _set_cron_array( $crons, $wp_error ); }
要使用` get_users
`函式獲取所有使用者列表,可以按照以下步驟進行:
1. 使用` get_users
`函式呼叫獲取使用者列表:
$users = get_users();
2. 您可以按需使用引數來過濾結果。例如,您可以通過角色、使用者ID、使用者登入名等過濾使用者列表。以下是一個根據使用者角色為過濾條件的示例:
$users = get_users( array( 'role' => 'subscriber' // 將角色名稱替換為您要過濾的角色 ) );
在上述示例中,將` role
`引數設定為所需的角色名稱來過濾使用者列表。
3. 您可以使用迴圈遍歷獲取的使用者列表,並訪問每個使用者的屬性。例如,以下示例將顯示每個使用者的使用者名稱和電子郵件地址:
foreach( $users as $user ) { echo '使用者名稱:' . $user->user_login . ', 電子郵件:' . $user->user_email . ; }
在上述示例中,通過` $user->user_login
`和` $user->user_email
`訪問每個使用者的使用者名稱和電子郵件地址。
請注意,` get_users
`函式預設返回所有使用者,並可以根據需要使用更多引數進行過濾。您可以參閱WordPress官方文件中的` get_users
`函式文件,瞭解更多可用引數和用法示例。
總結起來,使用` get_users
`函式獲取所有使用者列表的步驟是:
get_users
`函式獲取使用者列表。在WordPress中,可以使用WP_PLUGIN_DIR和WP_PLUGIN_URL常量來定義外掛的目錄路徑和URL。
1. `WP_PLUGIN_DIR`:這是一個常量,用於定義外掛的目錄路徑(檔案系統路徑)。您可以使用以下程式碼在外掛檔案中訪問該常量:
$plugin_dir = WP_PLUGIN_DIR . '/your-plugin-folder/';
在上述程式碼中,將"your-plugin-folder"替換為您外掛的實際資料夾名稱。使用該常量,您可以獲取外掛檔案的完整路徑。
2. `WP_PLUGIN_URL`:這是一個常量,用於定義外掛的URL(用於在網頁上訪問外掛檔案)。以下是一個使用該常量的示例:
$plugin_url = WP_PLUGIN_URL . '/your-plugin-folder/';
同樣,請將"your-plugin-folder"替換為您外掛的實際資料夾名稱。使用該常量,您可以獲取外掛在網頁上的完整URL。
請注意,`WP_PLUGIN_DIR`和`WP_PLUGIN_URL`常量在WordPress版本2.6之後引入。從WordPress 5.5版本開始,這兩個常量被標記為過時(deprecated),因為WordPress更傾向於使用新的外掛檔案結構。如果您正在開發新外掛,建議使用新的外掛檔案結構和相關函式。
在新的外掛檔案結構中,可以使用以下函式來獲取外掛的目錄路徑和URL:
- `plugin_dir_path()`:獲取外掛目錄路徑。
- `plugin_dir_url()`:獲取外掛URL。
這些函式會自動將外掛的版本、多站點和SSL等考慮因素納入計算。
總結起來,使用`WP_PLUGIN_DIR`和`WP_PLUGIN_URL`常量定義外掛的目錄和URL的方法是:
$plugin_dir = WP_PLUGIN_DIR . '/your-plugin-folder/'; $plugin_url = WP_PLUGIN_URL . '/your-plugin-folder/';
但請注意,這兩個常量已被標記為過時,建議使用新的外掛檔案結構和相關函式來獲取外掛的路徑和URL。
使用PHP在WordPress中新增自定義功能可以通過以下方式實現:
下面是一個實操示例。
要在WordPress中新增自定義功能,可以按照以下步驟使用PHP編寫並新增自定義功能:
// 新增自定義功能示例 // 1. 建立自定義短程式碼 function custom_shortcode() { return '這是我的自定義短程式碼內容'; } add_shortcode('custom', 'custom_shortcode'); // 2. 自定義小工具 function custom_widget() { echo '這是我的自定義小工具內容'; } register_widget('custom_widget'); // 3. 自定義選單 function custom_menu() { register_nav_menu('custom-menu', '自定義選單'); } add_action('after_setup_theme', 'custom_menu'); // 4. 自定義頁面模板 function custom_page_template() { /* Template Name: 自定義模板 */ // 自定義模板的內容和樣式 }
請注意,修改主題檔案可以在主題更新時丟失,因此建議在進行任何更改之前備份functions.php檔案。此外,為避免不必要的錯誤和衝突,建議在新增自定義功能前先了解WordPress開發文件和最佳實踐,以確保正確、安全地實現所需的自定義功能。
使用 do_action
函式可以觸發一個鉤子函式。do_action
函式的引數與要觸發的鉤子函式的引數相同。
例如,觸發save_post鉤子函式的程式碼如下:
do_action( 'save_post', $post_ID, $post );
這裡,$post_ID
和 $post
是傳遞給鉤子函式的引數。
使用 wp_get_current_user
獲取當前登入使用者的資訊:
$current_user = wp_get_current_user(); // 獲取當前使用者的ID $user_id = $current_user->ID; // 獲取當前使用者的使用者名稱 $user_login = $current_user->user_login; // 獲取當前使用者的郵箱 $user_email = $current_user->user_email; // 獲取當前使用者的顯示名稱 $display_name = $current_user->display_name;