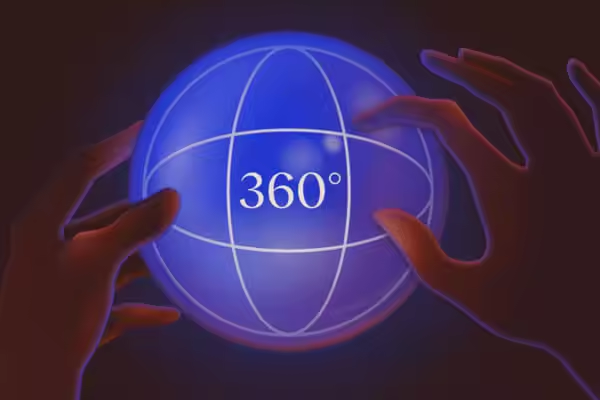
wp_set_object_terms ( $object_id, $terms, $taxonomy, $append = false )
wp_set_object_terms: 這個函式用來設定與一個物件相關的術語,例如一個文章或一個自定義文章型別。它接受物件的ID,術語ID的陣列,術語的分類法,以及更新物件相關的現有術語的各種選項。
建立術語和分類學關係。
將一個物件(文章、連結等)與一個術語和分類學型別聯絡起來。如果術語和分類法關係不存在,則建立該關係。如果術語不存在,則建立一個術語(使用lug)。
關係意味著該術語被歸入分類法中或屬於分類法。一個術語沒有意義,除非通過定義它存在於哪個分類法之下,給它提供背景。
function wp_set_object_terms( $object_id, $terms, $taxonomy, $append = false ) { global $wpdb; $object_id = (int) $object_id; if ( ! taxonomy_exists( $taxonomy ) ) { return new WP_Error( 'invalid_taxonomy', __( 'Invalid taxonomy.' ) ); } if ( ! is_array( $terms ) ) { $terms = array( $terms ); } if ( ! $append ) { $old_tt_ids = wp_get_object_terms( $object_id, $taxonomy, array( 'fields' => 'tt_ids', 'orderby' => 'none', 'update_term_meta_cache' => false, ) ); } else { $old_tt_ids = array(); } $tt_ids = array(); $term_ids = array(); $new_tt_ids = array(); foreach ( (array) $terms as $term ) { if ( '' === trim( $term ) ) { continue; } $term_info = term_exists( $term, $taxonomy ); if ( ! $term_info ) { // Skip if a non-existent term ID is passed. if ( is_int( $term ) ) { continue; } $term_info = wp_insert_term( $term, $taxonomy ); } if ( is_wp_error( $term_info ) ) { return $term_info; } $term_ids[] = $term_info['term_id']; $tt_id = $term_info['term_taxonomy_id']; $tt_ids[] = $tt_id; if ( $wpdb->get_var( $wpdb->prepare( "SELECT term_taxonomy_id FROM $wpdb->term_relationships WHERE object_id = %d AND term_taxonomy_id = %d", $object_id, $tt_id ) ) ) { continue; } /** * Fires immediately before an object-term relationship is added. * * @since 2.9.0 * @since 4.7.0 Added the `$taxonomy` parameter. * * @param int $object_id Object ID. * @param int $tt_id Term taxonomy ID. * @param string $taxonomy Taxonomy slug. */ do_action( 'add_term_relationship', $object_id, $tt_id, $taxonomy ); $wpdb->insert( $wpdb->term_relationships, array( 'object_id' => $object_id, 'term_taxonomy_id' => $tt_id, ) ); /** * Fires immediately after an object-term relationship is added. * * @since 2.9.0 * @since 4.7.0 Added the `$taxonomy` parameter. * * @param int $object_id Object ID. * @param int $tt_id Term taxonomy ID. * @param string $taxonomy Taxonomy slug. */ do_action( 'added_term_relationship', $object_id, $tt_id, $taxonomy ); $new_tt_ids[] = $tt_id; } if ( $new_tt_ids ) { wp_update_term_count( $new_tt_ids, $taxonomy ); } if ( ! $append ) { $delete_tt_ids = array_diff( $old_tt_ids, $tt_ids ); if ( $delete_tt_ids ) { $in_delete_tt_ids = "'" . implode( "', '", $delete_tt_ids ) . "'"; $delete_term_ids = $wpdb->get_col( $wpdb->prepare( "SELECT tt.term_id FROM $wpdb->term_taxonomy AS tt WHERE tt.taxonomy = %s AND tt.term_taxonomy_id IN ($in_delete_tt_ids)", $taxonomy ) ); $delete_term_ids = array_map( 'intval', $delete_term_ids ); $remove = wp_remove_object_terms( $object_id, $delete_term_ids, $taxonomy ); if ( is_wp_error( $remove ) ) { return $remove; } } } $t = get_taxonomy( $taxonomy ); if ( ! $append && isset( $t->sort ) && $t->sort ) { $values = array(); $term_order = 0; $final_tt_ids = wp_get_object_terms( $object_id, $taxonomy, array( 'fields' => 'tt_ids', 'update_term_meta_cache' => false, ) ); foreach ( $tt_ids as $tt_id ) { if ( in_array( (int) $tt_id, $final_tt_ids, true ) ) { $values[] = $wpdb->prepare( '(%d, %d, %d)', $object_id, $tt_id, ++$term_order ); } } if ( $values ) { if ( false === $wpdb->query( "INSERT INTO $wpdb->term_relationships (object_id, term_taxonomy_id, term_order) VALUES " . implode( ',', $values ) . ' ON DUPLICATE KEY UPDATE term_order = VALUES(term_order)' ) ) { return new WP_Error( 'db_insert_error', __( 'Could not insert term relationship into the database.' ), $wpdb->last_error ); } } } wp_cache_delete( $object_id, $taxonomy . '_relationships' ); wp_cache_delete( 'last_changed', 'terms' ); /** * Fires after an object's terms have been set. * * @since 2.8.0 * * @param int $object_id Object ID. * @param array $terms An array of object term IDs or slugs. * @param array $tt_ids An array of term taxonomy IDs. * @param string $taxonomy Taxonomy slug. * @param bool $append Whether to append new terms to the old terms. * @param array $old_tt_ids Old array of term taxonomy IDs. */ do_action( 'set_object_terms', $object_id, $terms, $tt_ids, $taxonomy, $append, $old_tt_ids ); return $tt_ids; }
要使用` get_users
`函式獲取所有使用者列表,可以按照以下步驟進行:
1. 使用` get_users
`函式呼叫獲取使用者列表:
$users = get_users();
2. 您可以按需使用引數來過濾結果。例如,您可以通過角色、使用者ID、使用者登入名等過濾使用者列表。以下是一個根據使用者角色為過濾條件的示例:
$users = get_users( array( 'role' => 'subscriber' // 將角色名稱替換為您要過濾的角色 ) );
在上述示例中,將` role
`引數設定為所需的角色名稱來過濾使用者列表。
3. 您可以使用迴圈遍歷獲取的使用者列表,並訪問每個使用者的屬性。例如,以下示例將顯示每個使用者的使用者名稱和電子郵件地址:
foreach( $users as $user ) { echo '使用者名稱:' . $user->user_login . ', 電子郵件:' . $user->user_email . ; }
在上述示例中,通過` $user->user_login
`和` $user->user_email
`訪問每個使用者的使用者名稱和電子郵件地址。
請注意,` get_users
`函式預設返回所有使用者,並可以根據需要使用更多引數進行過濾。您可以參閱WordPress官方文件中的` get_users
`函式文件,瞭解更多可用引數和用法示例。
總結起來,使用` get_users
`函式獲取所有使用者列表的步驟是:
get_users
`函式獲取使用者列表。在WordPress中,可以使用WP_PLUGIN_DIR和WP_PLUGIN_URL常量來定義外掛的目錄路徑和URL。
1. `WP_PLUGIN_DIR`:這是一個常量,用於定義外掛的目錄路徑(檔案系統路徑)。您可以使用以下程式碼在外掛檔案中訪問該常量:
$plugin_dir = WP_PLUGIN_DIR . '/your-plugin-folder/';
在上述程式碼中,將"your-plugin-folder"替換為您外掛的實際資料夾名稱。使用該常量,您可以獲取外掛檔案的完整路徑。
2. `WP_PLUGIN_URL`:這是一個常量,用於定義外掛的URL(用於在網頁上訪問外掛檔案)。以下是一個使用該常量的示例:
$plugin_url = WP_PLUGIN_URL . '/your-plugin-folder/';
同樣,請將"your-plugin-folder"替換為您外掛的實際資料夾名稱。使用該常量,您可以獲取外掛在網頁上的完整URL。
請注意,`WP_PLUGIN_DIR`和`WP_PLUGIN_URL`常量在WordPress版本2.6之後引入。從WordPress 5.5版本開始,這兩個常量被標記為過時(deprecated),因為WordPress更傾向於使用新的外掛檔案結構。如果您正在開發新外掛,建議使用新的外掛檔案結構和相關函式。
在新的外掛檔案結構中,可以使用以下函式來獲取外掛的目錄路徑和URL:
- `plugin_dir_path()`:獲取外掛目錄路徑。
- `plugin_dir_url()`:獲取外掛URL。
這些函式會自動將外掛的版本、多站點和SSL等考慮因素納入計算。
總結起來,使用`WP_PLUGIN_DIR`和`WP_PLUGIN_URL`常量定義外掛的目錄和URL的方法是:
$plugin_dir = WP_PLUGIN_DIR . '/your-plugin-folder/'; $plugin_url = WP_PLUGIN_URL . '/your-plugin-folder/';
但請注意,這兩個常量已被標記為過時,建議使用新的外掛檔案結構和相關函式來獲取外掛的路徑和URL。
使用PHP在WordPress中新增自定義功能可以通過以下方式實現:
下面是一個實操示例。
要在WordPress中新增自定義功能,可以按照以下步驟使用PHP編寫並新增自定義功能:
// 新增自定義功能示例 // 1. 建立自定義短程式碼 function custom_shortcode() { return '這是我的自定義短程式碼內容'; } add_shortcode('custom', 'custom_shortcode'); // 2. 自定義小工具 function custom_widget() { echo '這是我的自定義小工具內容'; } register_widget('custom_widget'); // 3. 自定義選單 function custom_menu() { register_nav_menu('custom-menu', '自定義選單'); } add_action('after_setup_theme', 'custom_menu'); // 4. 自定義頁面模板 function custom_page_template() { /* Template Name: 自定義模板 */ // 自定義模板的內容和樣式 }
請注意,修改主題檔案可以在主題更新時丟失,因此建議在進行任何更改之前備份functions.php檔案。此外,為避免不必要的錯誤和衝突,建議在新增自定義功能前先了解WordPress開發文件和最佳實踐,以確保正確、安全地實現所需的自定義功能。
使用 do_action
函式可以觸發一個鉤子函式。do_action
函式的引數與要觸發的鉤子函式的引數相同。
例如,觸發save_post鉤子函式的程式碼如下:
do_action( 'save_post', $post_ID, $post );
這裡,$post_ID
和 $post
是傳遞給鉤子函式的引數。
使用 wp_get_current_user
獲取當前登入使用者的資訊:
$current_user = wp_get_current_user(); // 獲取當前使用者的ID $user_id = $current_user->ID; // 獲取當前使用者的使用者名稱 $user_login = $current_user->user_login; // 獲取當前使用者的郵箱 $user_email = $current_user->user_email; // 獲取當前使用者的顯示名稱 $display_name = $current_user->display_name;