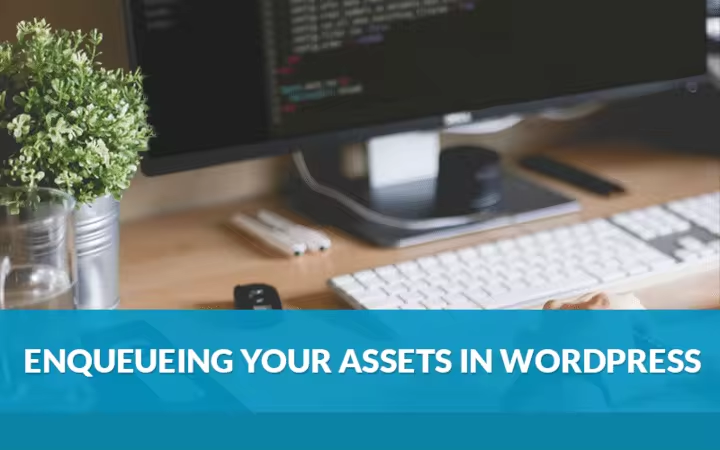
在WordPress中,不是簡單地將這些新增到標題中,您應該使用一種稱為入隊的方法,這是一種處理資產的標準化方式,具有管理依賴項的額外好處。在下面瞭解如何使用 wp_enqueue_scripts
.
排隊的工作原理
將指令碼或樣式排入佇列時需要執行兩個步驟。首先你註冊它——告訴WordPress它在那裡——然後你實際上將它排入佇列,最終將它輸出到標題中或在結束正文標籤之前。
有兩個步驟的原因與模組化有關。有時您會想讓WordPress知道某個資產,但您可能不想在每個頁面上都使用它。例如:如果您正在構建一個使用Javascript的自定義相簿簡碼,您實際上只需要在使用簡碼時載入JS – 可能不是在每個頁面上。
實現這一點的方法是首先註冊指令碼,只有在顯示簡碼時才真正將其排入佇列。
使用wp_enqueue_scripts入隊基礎知識
要在前端排隊指令碼和樣式,您需要使用wp_enqueue_scripts
鉤子。在該掛鉤的函式可以使用wp_register_script()
,wp_enqueue_script()
,wp_register_style()
和wp_enqueue_style()
功能。
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_register_style( 'custom-gallery', plugins_url( '/css/gallery.css' , __FILE__ ) );
wp_register_script( 'custom-gallery', plugins_url( '/js/gallery.js' , __FILE__ ) );
wp_enqueue_style( 'custom-gallery' );
wp_enqueue_script( 'custom-gallery' );
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_register_style( 'custom-gallery', plugins_url( '/css/gallery.css' , __FILE__ ) );
wp_register_script( 'custom-gallery', plugins_url( '/js/gallery.js' , __FILE__ ) );
wp_enqueue_style( 'custom-gallery' );
wp_enqueue_script( 'custom-gallery' );
}
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_register_style( 'custom-gallery', plugins_url( '/css/gallery.css' , __FILE__ ) );
wp_register_script( 'custom-gallery', plugins_url( '/js/gallery.js' , __FILE__ ) );
wp_enqueue_style( 'custom-gallery' );
wp_enqueue_script( 'custom-gallery' );
}
在上面的例子中,我在同一個函式中註冊和排隊資產,這有點多餘。實際上,您可以使用入隊函式立即註冊和入隊,方法是使用與註冊函式中相同的引數:
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_enqueue_style( 'custom-gallery', plugins_url( '/css/gallery.css' , __FILE__ ) );
wp_enqueue_script( 'custom-gallery', plugins_url( '/js/gallery.js' , __FILE__ ) );
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_enqueue_style( 'custom-gallery', plugins_url( '/css/gallery.css' , __FILE__ ) );
wp_enqueue_script( 'custom-gallery', plugins_url( '/js/gallery.js' , __FILE__ ) );
}
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_enqueue_style( 'custom-gallery', plugins_url( '/css/gallery.css' , __FILE__ ) );
wp_enqueue_script( 'custom-gallery', plugins_url( '/js/gallery.js' , __FILE__ ) );
}
如果我要將這兩個函式分開,我會通過在不同的鉤子中使用它們來實現。在一個真實的例子中,我們可以使用wp_enqueue_scripts
鉤子來註冊資產和短程式碼的函式來將它們排入佇列。
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_register_style( 'custom-gallery', plugins_url( '/css/gallery.css' , __FILE__ ) );
wp_register_script( 'custom-gallery', plugins_url( '/js/gallery.js' , __FILE__ ) );
add_shortcode( 'custom_gallery', 'custom_gallery' );
function custom_gallery( $atts ){
wp_enqueue_style( 'custom-gallery' );
wp_enqueue_script( 'custom-gallery' );
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_register_style( 'custom-gallery', plugins_url( '/css/gallery.css' , __FILE__ ) );
wp_register_script( 'custom-gallery', plugins_url( '/js/gallery.js' , __FILE__ ) );
}
add_shortcode( 'custom_gallery', 'custom_gallery' );
function custom_gallery( $atts ){
wp_enqueue_style( 'custom-gallery' );
wp_enqueue_script( 'custom-gallery' );
// Gallery code here
}
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_register_style( 'custom-gallery', plugins_url( '/css/gallery.css' , __FILE__ ) );
wp_register_script( 'custom-gallery', plugins_url( '/js/gallery.js' , __FILE__ ) );
}
add_shortcode( 'custom_gallery', 'custom_gallery' );
function custom_gallery( $atts ){
wp_enqueue_style( 'custom-gallery' );
wp_enqueue_script( 'custom-gallery' );
// Gallery code here
}
依賴管理
WordPress的排隊機制內建了對依賴項管理的支援,使用兩者wp_register_style()
和wp_register_script()
函式的第三個引數。如果您不需要將它們分開,您也可以立即使用排隊功能。
第三個引數是需要在當前資產入隊之前載入的已註冊指令碼/樣式陣列。我們上面的例子很可能依賴於jQuery,所以讓我們現在指定:
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_enqueue_script( 'custom-gallery', plugins_url( '/js/gallery.js' , __FILE__ ), array( 'jquery' ) );
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_enqueue_script( 'custom-gallery', plugins_url( '/js/gallery.js' , __FILE__ ), array( 'jquery' ) );
}
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_enqueue_script( 'custom-gallery', plugins_url( '/js/gallery.js' , __FILE__ ), array( 'jquery' ) );
}
我們不需要自己註冊或排隊jQuery,因為它已經是WordPress的一部分。您可以在Codex中找到WordPress中可用的指令碼和樣式列表。
如果您有自己的依賴項,則需要註冊它們,否則您的指令碼將無法載入。下面是一個例子:
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_enqueue_script( 'custom-gallery', plugins_url( '/js/gallery.js' , __FILE__ ), array( 'jquery' ) );
wp_enqueue_script( 'custom-gallery-lightbox', plugins_url( '/js/gallery-lightbox.js' , __FILE__ ), array( 'custom-gallery' ) );
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_enqueue_script( 'custom-gallery', plugins_url( '/js/gallery.js' , __FILE__ ), array( 'jquery' ) );
wp_enqueue_script( 'custom-gallery-lightbox', plugins_url( '/js/gallery-lightbox.js' , __FILE__ ), array( 'custom-gallery' ) );
}
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_enqueue_script( 'custom-gallery', plugins_url( '/js/gallery.js' , __FILE__ ), array( 'jquery' ) );
wp_enqueue_script( 'custom-gallery-lightbox', plugins_url( '/js/gallery-lightbox.js' , __FILE__ ), array( 'custom-gallery' ) );
}
假設第一個指令碼是畫廊,第二個指令碼是使影象在燈箱中開啟的擴充套件。請注意,即使我們的第二個指令碼依賴於jQuery,我們也不需要指定這一點,因為我們的第一個指令碼已經載入了jQuery。也就是說,宣告所有依賴項可能是個好主意,只是為了確保如果您忘記包含依賴項,不會有任何破壞。
WordPress 現在知道我們需要哪些指令碼,並且可以計算出需要將它們新增到頁面的順序。
每當您可以在頁尾中載入指令碼時,您都應該這樣做。這會增加明顯的頁面載入時間,並可以防止您的網站在載入指令碼時掛起,尤其是當它們包含AJAX呼叫時。
入隊機制可以使用第五個引數(第四個是可選版本號)向頁尾新增指令碼。如果我們稍微修改一下,上面的示例將載入頁尾中的指令碼。
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_enqueue_script( 'custom-gallery', plugins_url( '/js/gallery.js' , __FILE__ ), array( 'jquery' ), '1.0', true );
wp_enqueue_script( 'custom-gallery-lightbox', plugins_url( '/js/gallery-lightbox.js' , __FILE__ ), array( 'custom-gallery', 'jquery' ), '1.0', true );
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_enqueue_script( 'custom-gallery', plugins_url( '/js/gallery.js' , __FILE__ ), array( 'jquery' ), '1.0', true );
wp_enqueue_script( 'custom-gallery-lightbox', plugins_url( '/js/gallery-lightbox.js' , __FILE__ ), array( 'custom-gallery', 'jquery' ), '1.0', true );
}
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_enqueue_script( 'custom-gallery', plugins_url( '/js/gallery.js' , __FILE__ ), array( 'jquery' ), '1.0', true );
wp_enqueue_script( 'custom-gallery-lightbox', plugins_url( '/js/gallery-lightbox.js' , __FILE__ ), array( 'custom-gallery', 'jquery' ), '1.0', true );
}
指定true作為第五個引數會將指令碼放在頁尾中,使用false或省略引數將在頁首中載入內容。正如我之前提到的,只要有可能,就在頁尾中載入指令碼。
使用樣式註冊/入隊功能的第五個引數,您可以控制已定義指令碼的媒體型別(列印、螢幕、手持裝置等)。通過使用此引數,您可以將樣式的載入限制為特定的媒體型別,這是一個方便的小優化技巧。
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_register_style( 'custom-gallery-print', plugins_url( '/css/gallery.css' , __FILE__ ), array(), '1.0', 'print' );
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_register_style( 'custom-gallery-print', plugins_url( '/css/gallery.css' , __FILE__ ), array(), '1.0', 'print' );
}
add_action( 'wp_enqueue_scripts', 'my_plugin_assets' );
function my_plugin_assets() {
wp_register_style( 'custom-gallery-print', plugins_url( '/css/gallery.css' , __FILE__ ), array(), '1.0', 'print' );
}
有關可以使用的媒體型別的完整列表,請檢視CSS規範。
小結
對資產進行排隊是一種強大的處理方式。它為您和其他外掛/主題製作者提供了對整個系統的更多控制,並使依賴管理脫離您的手。
如果這還不夠,這是新增資源的方式,如果您不使用此方法,許多主題市場和WordPress外掛庫本身將不會批准您的工作。
評論留言